SSD1306OLED
A hardware driver for the Adafruit 128x32 OLED and Adafruit 128x64 OLED panels, which are based on the Solomon SSD1306 controller. The OLEDs communicate over any I²C bus.
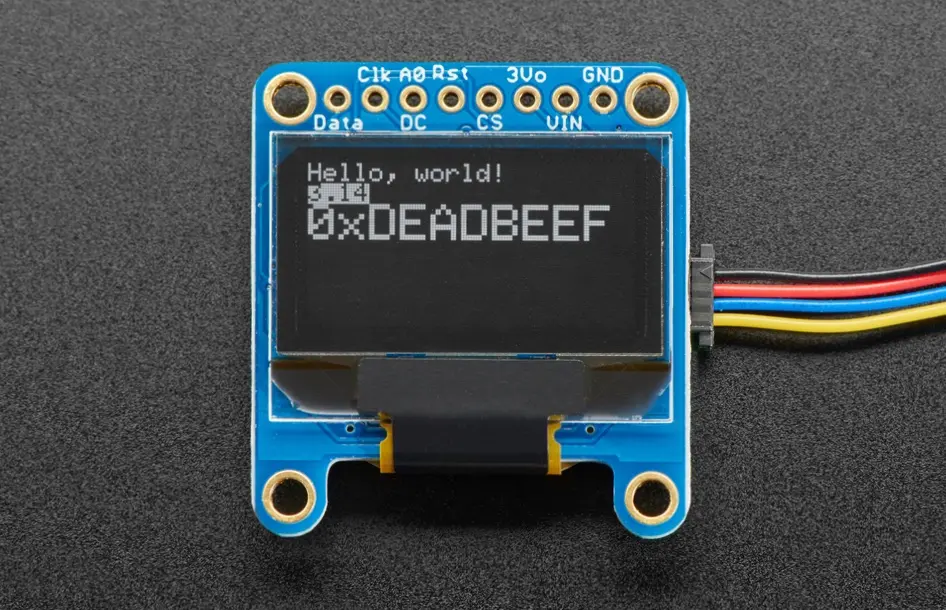
Adafruit 128x32 OLED. Image © Adafruit
Compatibility
SSD1306OLED
is compatible with CircuitPython and MicroPython.
Character Set
The driver contains a full, proportionally spaced Ascii character set.
I²C Addressing
The displays have the following default I²C addresses:
128x32 —
0x3C
128x64 —
0x3D
The first of these is the constructor default, so you will need to pass in the second value if you are using the larger panel. You will need to pass in an alternative address if you have changed it at the board level.
Source Code
You can find the source code on GitHub.
Class Constructor
- class SSD1306OLED(reset_pin, i2c, address=0x3C, height=32, width=128)
To instantiate an SSD1306OLED object pass the reset pin and I²C bus to which the display is connected, and, optionally, its I²C address, pixel height and pixel width.
The passed reset pin and I²C bus must be configured before the SSD1306OLED object is created.
- Parameters:
reset_pin (Pin object) – The GPIO pin connected to the OLED’s reset pin.
i2c (I²C bus object) – The I²C bus to which the display is connected.
address (Integer) – An optional I²C address. Default:
0x3C
.width (Integer) – The width of the display in pixels. Default: 128.
height (Integer) – The height of the display in pixels. Default: 32.
Examples
# CircuitPython
import board
import busio
from ssd1306 import SSD1306
# Set up I2C
i2c = busio.I2C(board.SCL, board.SDA)
# Set up the RST pin
reset = digitalio.DigitalInOut(board.D7)
reset.direction = digitalio.Direction.OUTPUT
# Set up OLED display using the default settings
display = SSD1306OLED(reset, i2c)
# MicroPython
from machine import I2C, Pin
from ssd1306 import SSD1306
# Set up I2C on Raspberry Pi Pico
i2c = I2C(0, scl=Pin(9), sda=Pin(8))
# Set up the RST pin
reset = Pin(7, Pin.OUT)
# Set up OLED display using the default settings
display = SSD1306OLED(reset, i2c)
Class Methods
- SSD1306OLED.set_inverse(is_inverse=True)
This method is called to set the display to black-on-white (
True
) or white-on-black (False
).- Parameters:
is_inverse (Boolean) – Whether to invert the OLED’s pixels. Default:
True
.
- SSD1306OLED.move(x, y)
Move the cursor to the specified co-ordinates.
- Parameters:
x (Integer) – The X co-ordinate in pixels, in range 0-127.
y (Integer) – The Y co-ordinate in pixels, in range 0-31 or 0-63 depending on model.
- Returns:
The instance (
self
).
- SSD1306OLED.home()
Move the cursor to the home position: the top left of the screen
(0,0)
.- Returns:
The instance (
self
).
- SSD1306OLED.text(print_string, do_wrap=False)
Place the specified text (8px) at the current co-ordinates as set by move().
- Parameters:
print_string (String) – The text to display.
do_wrap (Boolean) – Should overflowing text wrap. Default:
False
.
- Returns:
The instance (
self
).
- SSD1306OLED.text_2x(print_string, do_wrap=False)
Place the specified double-size (16px) text at the current co-ordinates as set by move().
- Parameters:
print_string (String) – The text to display.
do_wrap (Boolean) – Should overflowing text wrap. Default:
False
.
- Returns:
The instance (
self
).
- SSD1306OLED.length_of_string(print_string)
Determine the length of a printable string in pixels.
Use this method to learn how much screen space the specified string will take. This can help you choose where to place the cursor to draw the text with text(), for example to centre the text.
This assumes standard-size (8px) characters and a single-pixel space between adjacent characters.
- Parameters:
print_string (String) – The text to measure.
- Returns:
The length of the string in pixels.
- SSD1306OLED.plot(x, y, colour=1)
This method sets or clears a single pixel, specified by its co-ordinates, on the display. It does not update the screen — call draw() to do so.
By default,
plot()
uses the current ink colour: white for a white-on-black display, or black for black-on-white (see set_inverse()), but you can set the ink colour to clear the pixel instead. Pass1
for the foreground colour (the default) or0
for the background colour.- Parameters:
x (Integer) – The X co-ordinate in pixels, in range 0-127.
y (Integer) – The Y co-ordinate in pixels, in range 0-31 or 0-63 depending on model.
colour (Integer) – The ink colour, 1 (set) or 0 (clear). Default: 1.
- Returns:
The instance (
self
).
Example
# Put dots in the corners
display.plot(0,0).plot(0,31)
display.plot(127,0).plot(127,31)
display.draw()
- SSD1306OLED.line(x, y, tox, toy, thick=1, colour=1)
This method draws a line between the co-ordinates
(x,y)
and(tox,toy)
of the required thickness. If no thickness is specified the method defaults to a single pixel. If no ink colour is specified, the method defaults to the current foreground colour.line()
returns a reference to the driver instance in order to allow command chaining. It does not update the screen — call draw() to do so.- Parameters:
x (Integer) – The line’s start X co-ordinate in pixels, in range 0-127.
y (Integer) – The line’s start Y co-ordinate in pixels, in range 0-31 or 0-63 depending on model.
tox (Integer) – The line’s end X co-ordinate in pixels, in range 0-127.
toy (Integer) – The line’s endY co-ordinate in pixels, in range 0-31 or 0-63 depending on model.
thick (Integer) – The line thickness in pixels. Default: 1.
colour (Integer) – The ink colour, 1 (set) or 0 (clear). Default: 1.
- Returns:
The instance (
self
).
Example
# Draw two diagonal lines
display.line(0,0,127,31).line(127,0,0,31).draw()
- SSD1306OLED.circle(x, y, radius, colour=1, fill=False)
This method draws a circle centred on the co-ordinates
(x,y)
and with the specified radius. If no ink colour is specified, the method defaults to the current foreground colour. You can also opt to passTrue
into thefill
parameter to create a solid circle; otherwise an outline will be drawn.circle()
does not update the screen — call draw() to do so.- Parameters:
x (Integer) – The X co-ordinate of the circle’s centre in pixels, in range 0-127.
y (Integer) – The Y co-ordinate of the circle’s centre in pixels, in range 0-31 or 0-63 depending on model.
radius (Integer) – The circle’s radius in pixels.
colour (Integer) – The ink colour, 1 (set) or 0 (clear). Default: 1.
fill (Bool) – Should the circle be solid (
True
) or outline (False
). Default:False
.
- Returns:
The instance (
self
).
Example
# Draw two filled circles side by side
display.circle(47, 16, 14, 1, True).circle(81, 16, 14, 1, True).draw()
- SSD1306OLED.rect(x, y, width, height, colour=1, fill=False)
This method draws a rectangle between
(x,y)
and(x + width, y + height)
.If no ink colour is specified, the method defaults to the current foreground colour. You can opt to pass
True
into thefill
parameter to create a solid circle; otherwise an outline will be drawn.- Parameters:
x (Integer) – The rectangle’s start X co-ordinate in pixels, in range 0-127.
y (Integer) – The rectangle’s start Y co-ordinate in pixels, in range 0-31 or 0-63 depending on model.
width (Integer) – The rectangle’s width in pixels.
height (Integer) – The rectangle’s height in pixels.
colour (Integer) – The ink colour, 1 (set) or 0 (clear). Default: 1.
fill (Bool) – Should the rectangle be solid (
True
) or outline (False
). Default:False
.
- Returns:
The instance (
self
).
- SSD1306OLED.clear()
Clear the driver’s graphics buffer. It does not update the screen — call draw() to do so.
Example
display.clear().text("Hello, World!").draw()
- SSD1306OLED.draw()
Write the contents of the driver’s buffer to the display itself.
Example
# Draw a diagonal line
display.line(0,0,127,31).draw()